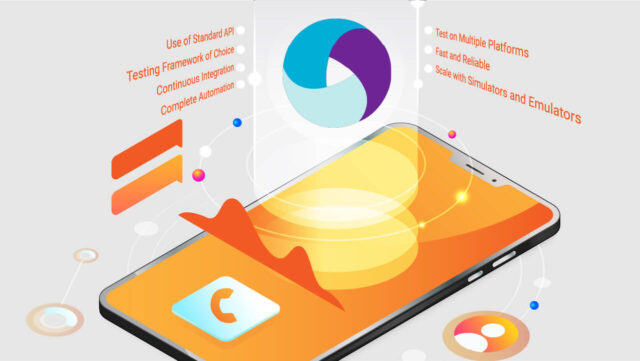
Appium is a popular open-source test automation framework for mobile applications. Appium Automation allows you to automate tests across multiple platforms like iOS, Android, and Windows using a single API. To run Appium tests, you need access to physical mobile devices or emulators/simulators.
Managing a test lab with multiple real devices can be time-consuming and challenging to scale. A better approach is to use an AI-powered test orchestration and execution platform like LambdaTest for cloud-based Appium automation.
With LambdaTest, you can whip your mobile app testing into shape. Their online cloud lets you buckle up and ride Appium automation at full gallop across thousands of devices. No need to bother wrangling physical devices yourself or hitting the dusty trail. LambdaTest has you covered faster than a pig can squeal!
LambdaTest provides instant access to a highly scalable cloud grid of thousands of real mobile devices, preconfigured for Appium automation. This guide will walk you through the process of setting up Appium on LambdaTest from scratch.
Our objective is to get you up and running with a sample Java test script leveraging Appium on LambdaTest. By the end, you’ll be able to:
- Configure your test capabilities and initialize your first Appium session on LambdaTest
- Understand the desired capabilities required to define your Appium test configuration
- Run a simple sample Appium test script on LambdaTest and view the results
- Explore some of the advanced testing features offered by LambdaTest, like video recording, logs, network traffic, etc.
By following this guide, you’ll be able to kickstart your Appium test automation on LambdaTest’s cloud with minimal hassle.
Prerequisites to Get Started
To follow along with this tutorial, there are a few requirements you need to fulfill beforehand:
LambdaTest Credentials
First, you’ll need a LambdaTest account. If you don’t have one yet, you can sign up on the LambdaTest website. Registration is free. Once signed in, your LambdaTest username and access key can be found on your account profile page. These credentials will be used later to connect your test script to the LambdaTest cloud.
Appium Client Libraries
Make sure you have the latest Java client libraries installed on your machine for Selenium and Appium. These packages contain the bindings to automate Appium testing through Java code. You can get them from Maven central repo or as direct JAR file downloads.
Maven Build Tool
Maven is the most popular Java build automation tool and dependency manager. Download and install the latest Maven on your system if you don’t have it already. Detailed installation steps can be found on the official Maven website. On Linux/MacOS, Maven can also be installed easily using Homebrew or other package managers.
Once you fulfill these prerequisites, you’ll be all set to integrate and run Appium Java tests on the LambdaTest platform using your personal account. Let’s now look at the steps to configure your first test.
Tutorial To Set Up Appium Automation on LambdaTest
Uploading Apps
Before running your test, you need to upload your application binary to LambdaTest. This makes your app available on the cloud to access from the automation test.
LambdaTest supports both iOS (.ipa) and Android (.apk/.aab) app formats. You can upload your app either from your local system or via a publicly available URL.
To upload, we’ll use LambdaTest’s Rest API, which accepts cURL commands. The API call needs to be authenticated using your LambdaTest username and access key.
Here is an example cURL request to upload an app file from your local system:
curl -u “YOUR_LAMBDATEST_USERNAME:YOUR_LAMBDATEST_ACCESS_KEY”
-X POST “https://manual-api.lambdatest.com/app/upload/realDevice”
-F “appFile=@”/Users/macuser/Downloads/proverbial_android.apk””
-F “name=”proverbial_app””
In this sample, replace “YOUR_LAMBDATEST_USERNAME” and “YOUR_LAMBDATEST_ACCESS_KEY” with your actual credentials. Also, update the “appFile” path to point to your actual .ipa or .apk file.
Similarly, you can provide a publicly accessible URL to your app instead of uploading the file:
curl -u “YOUR_LAMBDATEST_USERNAME:YOUR_LAMBDATEST_ACCESS_KEY”
-X POST “https://manual-api.lambdatest.com/app/upload/realDevice”
-F “url=https://prod-mobile-artefacts.lambdatest.com/assets/docs/proverbial_android.apk”
-F “name=Proverbial_App”
Once uploaded successfully, you’ll get an app ID which can be used during test configuration. Now let’s look at building our sample test script.
Cloning the Sample Project
We’ve created a sample Appium Java project for you to try out on LambdaTest. Let’s clone this project to your local machine first.
Open your terminal/command prompt and run the following git command to clone the repository:
git clone https://github.com/LambdaTest/LT-appium-java
This will create a local copy of the sample project.
Now change the directory to the project folder:
cd LT-appium-java
The sample project contains a simple test script called `LTAppiumTest.java` which opens up a to-do app, adds a new to-do item, and validates if the item was added.
We’ll use this script to run our first test on LambdaTest. But before executing, we need to configure the desired capabilities and authentication for our LambdaTest account.
The required dependencies like Appium client, TestNG, etc., are already added to this project. We’ve also configured the build automation using Maven.
Setting up Authentication
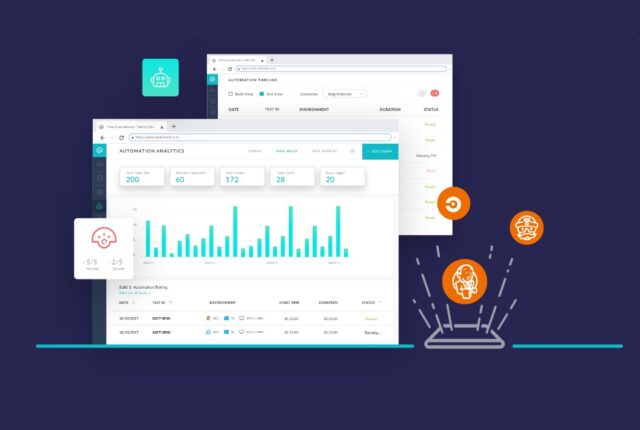
To run tests on LambdaTest, we need to provide the LambdaTest credentials in the test script for authentication.
Your LambdaTest username and access key can be obtained from your automation dashboard.
- Go to the LambdaTest platform and log in to your account.
- Navigate to the Automation dashboard.
- Your LambdaTest username and access key will be displayed here, which you can use in your scripts.
Rather than hard-coding credentials in the code, we’ll set them as environment variables on your machine:
On Linux/Mac
export LT_USERNAME=YOUR_LAMBDATEST_USERNAME
export LT_ACCESS_KEY=YOUR_LAMBDATEST_ACCESS_KEY
On Windows
set LT_USERNAME=YOUR_LAMBDATEST_USERNAME
set LT_ACCESS_KEY=YOUR_LAMBDATEST_ACCESS_KEY
Make sure to replace YOUR_LAMBDATEST_USERNAME and YOUR_LAMBDATEST_ACCESS_KEY with your actual credentials.
The script will automatically read these environment variables to connect to your LambdaTest account. This avoids exposing your credentials in the code.
Writing the Automation Script
Now that we have set up our authentication, let’s update the desired capabilities in the sample test script.
Desired capabilities define the test configuration on LambdaTest to match your application and test requirements.
The sample project contains a test class called `LTAppiumTest` with the capabilities and test logic.
Let’s look at the key capabilities we need to update:
- platformName – Operating system on which the test will run (e.g. iOS, Android)
- deviceName – Specific model or device name
- app – App URL uploaded earlier to LambdaTest
- platformVersion – OS version of the mobile device
- build – Optional build name for test execution
- name – Name of the test execution
Make sure to replace the placeholder values for app URL, platformName, deviceName etc, with your own.
The username and accessKey will be automatically read from the environment variables set earlier.
That completes the configuration required. The `LTAppiumTest` class also contains sample test logic to add a to-do item and validate it.
We are now all set to run the test on LambdaTest. The existing script has all the required Appium and TestNG frameworks configured.
Configuring Capabilities
The capabilities defined in the test script configure your test execution on LambdaTest as per your application and test requirements.
In the sample code, we are setting the capabilities in a `DesiredCapabilities` object.
For an Android test, the capabilities are defined as:
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(“deviceName”, “Galaxy S20”);
capabilities.setCapability(“platformVersion”, “11”);
capabilities.setCapability(“platformName”, “Android”);
// Other capabilities like build, test name, etc.
Similarly, for an iOS test:
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability(“deviceName”, “iPhone 12”);
caps.setCapability(“platformVersion”, “15”);
caps.setCapability(“platformName”, “iOS”);
// Other capabilities like build, test name, etc.
The `app` capability should contain your actual app URL uploaded previously.
LambdaTest provides a capabilities generator tool to help pick devices, platforms, and other configurations as per your need. With the capabilities updated, we are now ready to run our test on LambdaTest cloud. The existing test script will initialize the Appium driver based on these capabilities to start the test.
Executing the Test
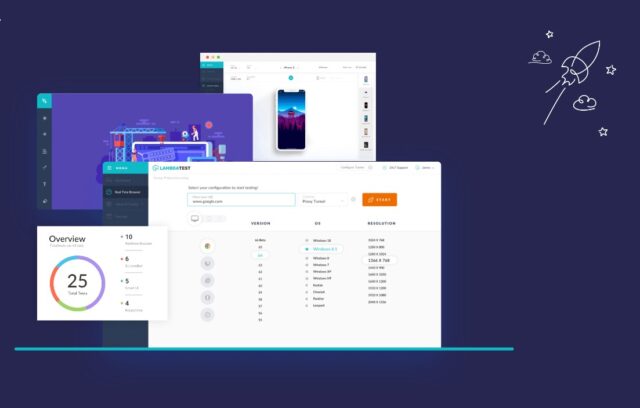
We are now ready to run our test script on LambdaTest!
The sample project uses Maven for build automation and dependency management.
First, let’s install the required dependencies:
mvn clean install
This will download the Selenium, Appium, and TestNG libraries needed to execute the test.
Now to trigger the test, use the following Maven commands:
For Android
mvn test -P android
For iOS
mvn test -P ios
The test will:
- Initialize the Appium driver on LambdaTest cloud using the configured capabilities.
- Install the app on the target device.
- Run the test logic to add a new to-do item and validate it.
- End the test and close the Appium session.
As the test runs, you will see the steps and assertions printed on the console.
Simultaneously, the test results will also be reflected on your LambdaTest automation dashboard.
You can access the detailed execution logs, videos, network logs, and other metadata to troubleshoot any issues.
With this, you have successfully run your first Appium test on the LambdaTest platform!
Advanced Testing Features of LambdaTest
LambdaTest comes with a host of advanced features to enhance your Appium automation testing and troubleshooting workflows.
Smart Test Orchestration
Intelligent test orchestration and scheduling to run your large test suites faster and more efficiently across the cloud device grid.
Real-time Debugging
View real-time console logs, video recordings, device streams, etc, to debug tests seamlessly without leaving the platform.
Smart Locators
Innovative locator suggestions through element presence detection and DOM analysis to fix broken locators.
HyperExecute
LambdaTest’s smart test execution engine for blazing fast parallel test runs while cutting infrastructure costs.
Automated Screenshots
Automatically capture screenshots before and after each test step to quickly pinpoint UI bugs and regressions.
Performance Metrics
In-depth performance analytics like CPU, memory, FPS, and network traffic to assess app performance across devices.
Integrations
End-to-end integration with your favorite tools like GitHub, Jira, Jenkins, Selenium and more for simplified collaboration.
On-Prem Deployment
Behind the firewall installation of LambdaTest grid on your infrastructure for enhanced security and data compliance.
These are just some of the key highlights of LambdaTest’s next-gen cloud testing platform to deliver unmatched quality, speed and scale.
Wrap-up
And that’s a wrap! In this tutorial, you learned how to:
- Set up and configure an Appium Java test script with desired capabilities
- Integrate the script with your LambdaTest account using the authentication
- Upload your app binary and generate an app URL
- Run the sample test on LambdaTest cloud and view the results
With this, you can now get started with automated Appium testing on LambdaTest’s reliable cloud-based infrastructure.
Some key highlights:
- LambdaTest provides online access to a scalable grid of thousands of real mobile devices. You get on-demand Appium automation testing without maintaining any lab.
- Smart test orchestration, scheduling, sharding, and console logs ensure your tests run faster and without hassles.
- Integration is quick through LambdaTest REST APIs and configuration via desired capabilities.
LambdaTest also publishes detailed guides and sample projects across multiple languages and frameworks to smoothen your Appium testing journey – be it Java, Python, NodeJS, PHP or others.
An interesting fact – LambdaTest offers 100 minutes of free Appium testing every month with a simple signup. Make the most of it to test your mobile apps at no cost!
In addition to mobile testing, LambdaTest provides online access to 3000+ browsers and operating systems for cross-browser compatibility testing of web apps. You can even manually test your websites and web apps online.
With a ton of features and a very generous free plan, LambdaTest can supercharge your dev testing workflows. Visit the website to learn more!